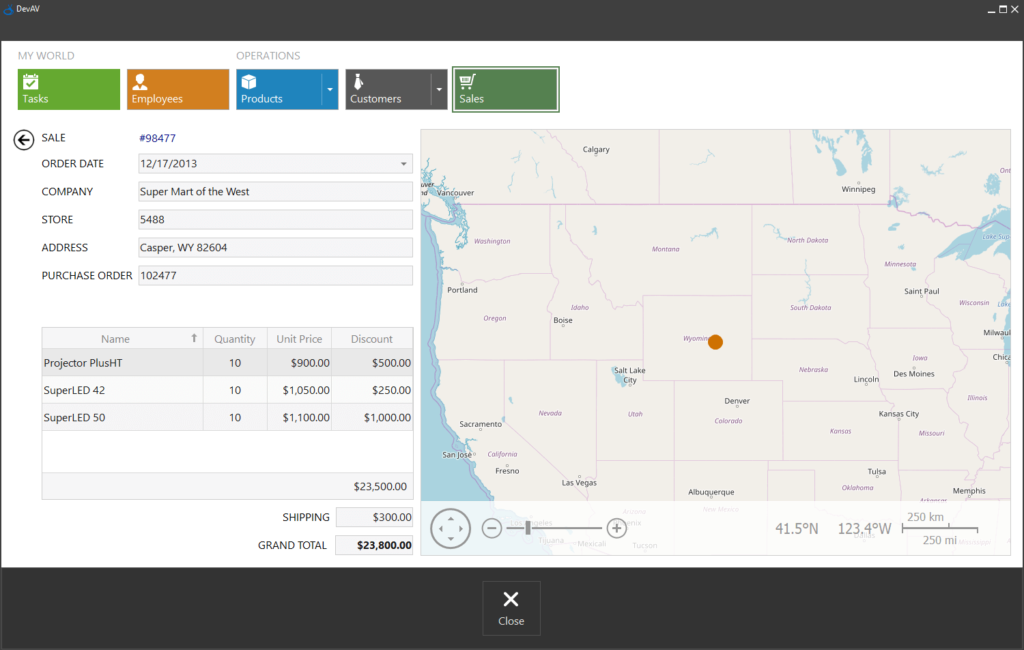
DevExpress is a well-known third-party component suite for Delphi that may be used to construct high-quality desktop and web applications. The suite comprises a wide range of visual and non-visual components that can substantially speed up the development process while also giving the user interface a clean and professional appearance and feel. In this post, we’ll look at how to use DevExpress in the Delphi programming language and present some useful examples.
Installing DevExpress in Delphi:
We must first install DevExpress before we can use it in our Delphi program. You can execute the installation file after downloading it from the DevExpress website. During the installation process, you will be prompted to choose which components to install. After installation, you must add the DevExpress components to the Delphi Component Palette.
Adding DevExpress Components to the Delphi Component Palette:
To add the DevExpress components to the Delphi Component Palette, follow these steps:
- Open Delphi and go to the Component menu
- Select “Install Packages”
- Click on the “Add” button and browse to the DevExpress installation folder
- Select the “DevExpress.VCL.x.y” package and click “Open”
- Click on the “OK” button to close the “Install Packages” dialog box
- The DevExpress components should now appear in the Delphi Component Palette.
Using DevExpress Components in Delphi:
Drag and drop a DevExpress component from the Component Palette onto your form to utilize it in your Delphi program. You can then modify the component’s properties in the Object Inspector. DevExpress components include a plethora of properties, events, and methods for customizing their behavior and appearance.
Here are some essential examples of DevExpress components that every Delphi programmer should know:
- Grid Control: The Grid Control is a powerful data grid that can display and edit data from a variety of data sources. It includes features such as sorting, filtering, grouping, and column customization. To use the Grid Control, you’ll need to connect it to a data source, such as a TDataSet or TClientDataSet component.
- Ribbon Control: The Ribbon Control is a modern user interface control that provides a tabbed toolbar with a customizable set of commands. It’s similar to the ribbon interface used in Microsoft Office applications. The Ribbon Control includes built-in support for themes and can be easily customized to match the look and feel of your application.
- Scheduler Control: The Scheduler Control is a component that can display and manage appointments and events. It includes features such as recurring appointments, resource allocation, and appointment reminders. You can customize the appearance and behavior of the Scheduler Control to meet your specific requirements.
- Tree List Control: The Tree List Control is a hierarchical data grid that can display data in a tree-like structure. It includes features such as sorting, filtering, and column customization. You can also customize the appearance of the Tree List Control, such as changing the background color of individual rows.
- Rich Edit Control: The Rich Edit Control is a component that provides advanced text editing and formatting capabilities. It supports features such as tables, images, and hyperlinks. You can also customize the appearance of the Rich Edit Control, such as changing the font size and style.
- Grid Control:
To display data in the Grid Control, you’ll need to connect it to a data source, such as a TDataSet or TClientDataSet component. Here’s an example of how to do that:
// Create a new TClientDataSet component
var
cdsData: TClientDataSet;
begin
cdsData := TClientDataSet.Create(Self);
cdsData.FieldDefs.Add(‘ID’, ftInteger, 0, True);
cdsData.FieldDefs.Add(‘Name’, ftString, 50, True);
cdsData.CreateDataSet;
cdsData.InsertRecord([1, ‘John’]);
cdsData.InsertRecord([2, ‘Jane’]);
// Connect the Grid Control to the TClientDataSet component
cxGrid1DBTableView1.DataController.DataSource := TDataSource.Create(Self);
cxGrid1DBTableView1.DataController.DataSource.DataSet := cdsData;
end;
- Ribbon Control:
To add commands to the Ribbon Control, you’ll need to create Ribbon Gallery Items. Here’s an example of how to create a Ribbon Gallery Item and add it to the Ribbon Control:
// Create a new Ribbon Gallery Item
var
item: TdxRibbonGalleryGroupItem;
begin
item := dxRibbon1Tab1.AddGalleryGroupItem(nil);
item.Caption := ‘Colors’;
item.GalleryImages := imgList;
item.GalleryOptions.ItemImageSize := Size(32, 32);
// Add items to the Ribbon Gallery Item
item.GalleryGroups.Add;
item.GalleryGroups[0].Caption := ‘Basic Colors’;
item.GalleryGroups[0].Items.Add;
item.GalleryGroups[0].Items[0].ImageIndex := 0;
item.GalleryGroups[0].Items[0].Caption := ‘Red’;
item.GalleryGroups[0].Items.Add;
item.GalleryGroups[0].Items[1].ImageIndex := 1;
item.GalleryGroups[0].Items[1].Caption := ‘Blue’;
end;
- Scheduler Control:
To add appointments to the Scheduler Control, you’ll need to create a TcxSchedulerStorage component and add the appointments to it. Here’s an example of how to do that:
// Create a new TcxSchedulerStorage component
var
storage: TcxSchedulerStorage;
begin
storage := TcxSchedulerStorage.Create(Self);
cxScheduler1.Storage := storage;
// Create a new appointment and add it to the storage
var
appointment: TcxSchedulerEvent;
appointment := cxScheduler1.Storage.CreateEvent;
appointment.Start := Now;
appointment.Finish := Now + EncodeTime(1, 0, 0, 0);
appointment.Subject := ‘Meeting’;
appointment.Location := ‘Conference Room’;
appointment.LabelColor := clYellow;
appointment.ReminderMinutesBeforeStart := 30;
cxScheduler1.Storage.AddEvent(appointment);
end;
- Tree List Control:
To display data in the Tree List Control, you’ll need to create a TcxTreeList component and add columns to it. Here’s an example of how to do that:
// Create a new TcxTreeList component
var
treeList: TcxTreeList;
begin
treeList := TcxTreeList.Create(Self);
treeList.Parent := Self;
treeList.Align := alClient;
// Add columns to the Tree List Control
var
column: TcxTreeListColumn;
column := treeList.CreateColumn;
column.Caption := ‘ID’;
column.DataBinding.ValueType := ‘Integer’;
column := treeList.CreateColumn; column.Caption := ‘Name’; column.DataBinding.ValueType := ‘String’;
// Add data to the Tree List Control
var rootNode, childNode: TcxTreeListNode;
rootNode := treeList.Root.Add;
rootNode.Values[0] := 1;
rootNode.Values[1] := ‘John’;
childNode := rootNode.AddChild;
childNode.Values[0] := 2;
childNode.Values[1] := ‘Jane’;
end;
- Chart Control:To display data in the Chart Control, you’ll need to create a TcxChartView component and add series to it. Here’s an example of how to do that:
“`delphi
// Create a new TcxChartView component
var
chartView: TcxChartView;
begin
chartView := TcxChartView.Create(Self);
chartView.Parent := Self;
chartView.Align := alClient;
// Add series to the Chart Control
var
series: TcxLineSeries;
series := chartView.CreateSeries(TcxLineSeries) as TcxLineSeries;
series.DataController.CreateSeries;
series.BeginUpdate;
try
series.ValuesDataBinding.FieldName := ‘Sales’;
series.ArgumentDataBinding.FieldName := ‘Month’;
series.DataController.DataSource := DataSource1;
finally
series.EndUpdate;
end;
// Populate the data source with data
DataSource1 := TDataSource.Create(Self);
DataSource1.DataSet := ADOQuery1;
ADOQuery1.Open;
end;
Finally, DevExpress is a comprehensive and robust component package that may dramatically improve the functionality and presentation of your Delphi projects. You’ll be well on your way to becoming adept in utilizing DevExpress in Delphi programming language if you follow the steps mentioned above and explore the main examples supplied.
Thanks for the good writeup. It actually was once a entertainment account it.
Glance complicated to more introduced agreeable from you!
However, how could we communicate?
Feel free to visit my web-site – nordvpn coupons inspiresensation (tinyurl.com)
You actually make it appear really easy along with your presentation however I in finding this matter to be actually
one thing which I think I might by no means understand.
It seems too complex and extremely large for me.
I am having a look forward in your subsequent submit, I’ll
attempt to get the hold of it!
my page … nordvpn Coupons Inspiresensation
We absolutely love your blog and find many of your post’s
to be exactly I’m looking for. Does one offer guest writers to write content for you personally?
I wouldn’t mind publishing a post or elaborating
on some of the subjects you write about here.
Again, awesome weblog!
Look at my blog :: nordvpn coupons inspiresensation
Pretty nice post. I just stumbled upon your weblog and wanted to
say that I have truly enjoyed browsing your blog posts.
In any case I’ll be subscribing to your feed and I hope you write again very soon!
Here is my web blog: nordvpn coupons inspiresensation; http://ourl.in,
350fairfax nordvpn
It’s hard to come by educated people on this topic, but you sound like
you know what you’re talking about! Thanks
Feel free to surf to my web-site – nord vpn promo
Definitely imagine that which you said. Your favourite reason appeared to be on the net the easiest thing to take into account of. I say to you, I certainly get irked at the same time as other folks consider worries that they plainly don’t know about. You managed to hit the nail upon the top and also outlined out the entire thing with no need side effect , other people can take a signal. Will likely be back to get more. Thanks
I discovered your blog site on google and check a few of your early posts. Continue to keep up the very good operate. I just additional up your RSS feed to my MSN News Reader. Seeking forward to reading more from you later on!…
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Thank you for the auspicious writeup. It in fact was a amusement account it. Look advanced to more added agreeable from you! However, how can we communicate?
The very root of your writing while sounding reasonable in the beginning, did not sit perfectly with me personally after some time. Somewhere throughout the sentences you actually were able to make me a believer unfortunately just for a very short while. I however have got a problem with your leaps in assumptions and you might do well to help fill in those breaks. When you actually can accomplish that, I will undoubtedly be amazed.
Your articles are extremely helpful to me. May I ask for more information? http://www.kayswell.com
Valuable information. Lucky me I found your web site by accident, and I am shocked why this accident didn’t happened earlier! I bookmarked it.
Lovely website! I am loving it!! Will be back later to read some more. I am taking your feeds also.
You made some first rate factors there. I looked on the internet for the problem and found most people will associate with with your website.
Just want to say your article is as astonishing. The clearness in your post is just spectacular and i can assume you’re an expert on this subject. Fine with your permission allow me to grab your feed to keep updated with forthcoming post. Thanks a million and please keep up the enjoyable work.
Generally I do not learn post on blogs, however I wish to say that this write-up very compelled me to take a look at and do so! Your writing style has been surprised me. Thank you, very great article.
These days of austerity and also relative panic about getting debt, a lot of people balk against the idea of making use of a credit card in order to make acquisition of merchandise or maybe pay for a holiday, preferring, instead to rely on the actual tried and also trusted means of making payment – hard cash. However, if you possess cash available to make the purchase entirely, then, paradoxically, that is the best time to be able to use the credit card for several good reasons.
Thank you for providing me with these article examples. May I ask you a question? http://www.ifashionstyles.com
The articles you write help me a lot and I like the topic http://www.kayswell.com
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Hi to all, how is all, I think every one is getting more from this web site,
and your views are nice designed for new people.
my blog eharmony special coupon code 2025
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
I wanted to thank you for this great read!! I definitely enjoying every little bit of it I have you bookmarked to check out new stuff you post…
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Very nice post. I simply stumbled upon your blog and wished to mention that
I have really enjoyed surfing around your blog posts.
After all I’ll be subscribing on your feed and I’m
hoping you write once more soon!
my web site … vpn
Thanks for posting. I really enjoyed reading it, especially because it addressed my problem. http://www.kayswell.com It helped me a lot and I hope it will help others too.
Great beat ! I would like to apprentice while you amend your web site, http://www.ifashionstyles.com how could i subscribe for a blog site? The account helped me a acceptable deal. I had been a little bit acquainted of this your broadcast provided bright clear concept
Sustain the excellent work and producing in the group! http://www.kayswell.com
Thank you for your articles. http://www.kayswell.com They are very helpful to me. Can you help me with something?
Your article helped me a lot, is there any more related content? Thanks!
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Thank you for writing this post! http://www.goodartdesign.com
It抯 really a nice and helpful piece of information. I抦 glad that you just shared this helpful information with us. Please stay us up to date like this. Thank you for sharing.
You helped me a lot with this post. http://www.kayswell.com I love the subject and I hope you continue to write excellent articles like this.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
You helped me a lot by posting this article and I love what I’m learning. http://www.hairstylesvip.com
Thank you for your articles. I find them very helpful. Could you help me with something? http://www.ifashionstyles.com
This site was… how do I say it? Relevant!! Finally I’ve found something that helped me.
Many thanks! gamefly 3 month free trial https://tinyurl.com/2cab6g88
May I request more information on the subject? http://www.kayswell.com All of your articles are extremely useful to me. Thank you!
I’m so in love with this. You did a great job!! http://www.kayswell.com
I discovered your weblog web site on google and examine a couple of of your early posts. Proceed to keep up the superb operate. I just further up your RSS feed to my MSN News Reader. Searching for ahead to studying more from you in a while!?
I want to thank you for your assistance and this post. It’s been great. http://www.kayswell.com