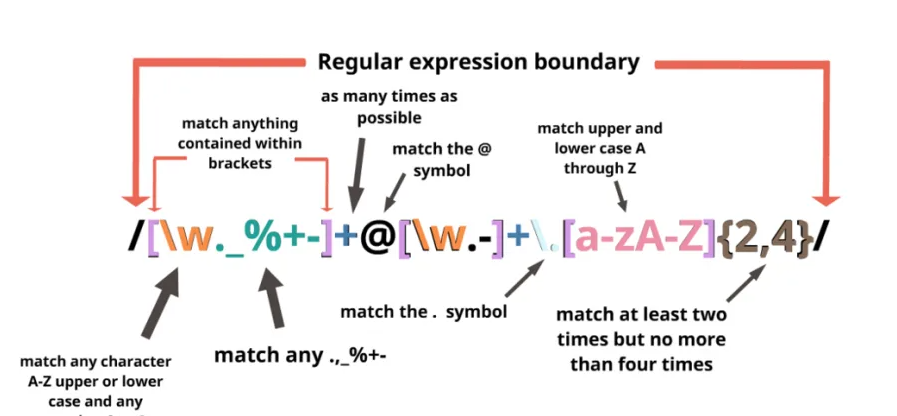
Regular expressions, commonly known as RegExpr, are powerful tools for pattern matching and manipulating strings. In the world of Delphi, leveraging RegExpr can greatly enhance your coding efficiency and simplify complex string operations. In this blog post, we’ll explore the basics of RegExpr in Delphi and create a library of useful functions that will make your coding experience even more enjoyable.
What are Regular Expressions? Regular expressions are sequences of characters that define a search pattern. They provide a concise and flexible means of searching, extracting, and replacing specific strings within larger bodies of text. RegExprs consist of both literal characters and special metacharacters that define the rules and patterns for the matching process.
Using Regular Expressions in Delphi: Delphi provides native support for RegExpr through the TRegEx
class in the RegularExpressions
unit. To start using RegExpr in your Delphi project, follow these steps:
Step 1: Import the required unit:
uses
RegularExpressions;
Step 2: Create an instance of the TRegEx
class:
var
RegEx: TRegEx;
begin
RegEx := TRegEx.Create;
Step 3: Utilize the various methods available: Delphi’s TRegEx
class provides a wide range of methods to work with regular expressions, such as IsMatch
, Replace
, Split
, and more. Let’s take a look at a few examples:
// Example 1: Check if a string matches a pattern
if RegEx.IsMatch('Hello, world!', 'Hello.*') then
ShowMessage('Pattern matched!')
else
ShowMessage('Pattern not found.');
// Example 2: Replace all occurrences of a pattern in a string
var
InputString, Pattern, Replacement: string;
begin
InputString := 'Hello, John! How are you, John?';
Pattern := 'John';
Replacement := 'Peter';
Result := RegEx.Replace(InputString, Pattern, Replacement);
ShowMessage(Result);
Creating a Library of Useful Functions: To further enhance your RegExpr capabilities in Delphi, let’s create a library of functions that encapsulate commonly used regular expression patterns. Here’s an example of a few functions you can include:
unit RegExprLibrary;
interface
uses
RegularExpressions, System.SysUtils;
type
TRegExprLibrary = class
private
FRegEx: TRegEx;
public
constructor Create;
function IsValidEmail(const Email: string): Boolean;
function ExtractNumbers(const Input: string): string;
function RemoveHTMLTags(const Input: string): string;
function IsValidIPAddress(const IPAddress: string): Boolean;
function ExtractURLs(const Input: string): TArray<string>;
// Add more methods as per your requirements
end;
implementation
constructor TRegExprLibrary.Create;
begin
FRegEx := TRegEx.Create;
end;
function TRegExprLibrary.IsValidEmail(const Email: string): Boolean;
const
EmailPattern = '^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}$';
begin
Result := FRegEx.IsMatch(Email, EmailPattern);
end;
function TRegExprLibrary.ExtractNumbers(const Input: string): string;
const
NumberPattern = '\d+';
var
Matches: TMatchCollection;
Match: TMatch;
I: Integer;
begin
Matches := FRegEx.Matches(Input, NumberPattern);
Result := '';
for I := 0 to Matches.Count - 1 do
begin
Match := Matches[I];
Result := Result + Match.Value;
if I < Matches.Count - 1 then
Result := Result + ', ';
end;
end;
function TRegExprLibrary.RemoveHTMLTags(const Input: string): string;
const
HTMLTagPattern = '<.*?>';
begin
Result := FRegEx.Replace(Input, HTMLTagPattern, '');
end;
function TRegExprLibrary.IsValidIPAddress(const IPAddress: string): Boolean;
const
IPAddressPattern = '^((25[0-5]|2[0-4]\d|[01]?\d\d?)\.){3}(25[0-5]|2[0-4]\d|[01]?\d\d?)$';
begin
Result := FRegEx.IsMatch(IPAddress, IPAddressPattern);
end;
function TRegExprLibrary.ExtractURLs(const Input: string): TArray<string>;
const
URLPattern = '((https?|ftp):\/\/)?([\da-z\.-]+)\.([a-z\.]{2,6})([\/\w \.-]*)*\/?';
var
Matches: TMatchCollection;
I: Integer;
begin
Matches := FRegEx.Matches(Input, URLPattern);
SetLength(Result, Matches.Count);
for I := 0 to Matches.Count - 1 do
Result[I] := Matches[I].Value;
end;
end.
These implementations demonstrate how the functions can be used with regular expressions in various scenarios:
IsValidEmail
: Validates if a given string is a valid email address.ExtractNumbers
: Extracts all numbers from a string and returns them as a comma-separated string.RemoveHTMLTags
: Removes all HTML tags from a string.IsValidIPAddress
: Validates if a given string is a valid IP address.ExtractURLs
: Extracts all URLs from a string and returns them as an array.
Feel free to modify these implementations or add more functions based on your requirements.
Remember to import the RegularExpressions
unit and take advantage of the various methods provided by TRegEx
to unleash the full potential of regular expressions in Delphi.
Happy coding!