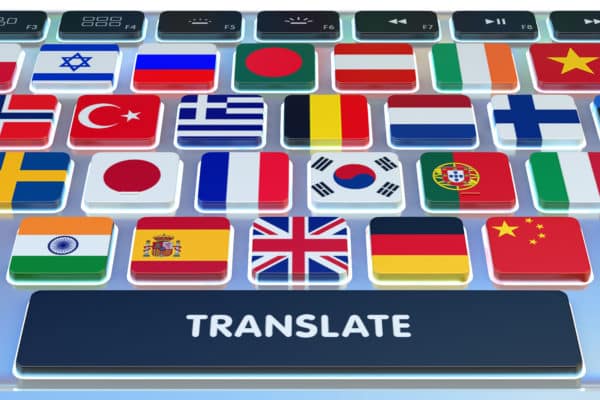
Using multilanguage in Delphi without 3rd party plugins is actually quite simple. Here are the steps you can follow:
Step 1: Create a resource string file
Create a new text file and save it with a .res extension. This file will contain all the strings that you want to translate. Here’s an example:
STRINGTABLE
{
101, "Hello, World!"
102, "Goodbye, World!"
}
In this example, we have two strings: “Hello, World!” and “Goodbye, World!”. The numbers before each string (101 and 102) are the resource IDs. These IDs will be used later in the Delphi code to access the strings.
Step 2: Add the resource file to your project
In Delphi, go to Project > Add to Project > Resource file and select the .res file you just created.
Step 3: Add the TMultiLang component to your form
In the Delphi IDE, go to the Component Palette and find the TMultiLang component. Drag and drop it onto your form.
Step 4: Set the resource file and default language
Select the TMultiLang component on your form and go to the Object Inspector. Set the ResourceFile property to the name of your .res file (without the .res extension). For example, if your file is called “strings.res”, set ResourceFile to “strings”.
Next, set the DefaultLanguage property to the language you want to use as the default. For example, if you want to use English as the default language, set DefaultLanguage to “en”.
Step 5: Add the strings to your form
Select the TMultiLang component on your form and go to the Object Inspector. Click on the Strings property and add the resource IDs and their corresponding translations. For example:
101=Hello, World!
102=Goodbye, World!
Step 6: Access the strings in the code
To access the translated strings in code, use the TMultiLang.GetText method. For example:
Label1.Caption := MultiLang1.GetText(101);
This code will set the caption of Label1 to the translated string with the resource ID 101.
Here’s the full code for a simple Delphi form that uses multilanguage:
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, MultiLang;
type
TForm1 = class(TForm)
MultiLang1: TMultiLang;
Label1: TLabel;
Button1: TButton;
procedure Button1Click(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure TForm1.Button1Click(Sender: TObject);
begin
Label1.Caption := MultiLang1.GetText(101);
end;
end.
The .dfm file for this form should contain the TMultiLang component, and the Strings property should be set to the translations you want to use.
To use multilanguage pictures in Delphi using TMultiResBitmap, you can follow these steps:
Step 1: Create a resource file for each image
Create a separate resource file for each image you want to use. Each file should contain the same image in different sizes. For example, if you have an image called “logo.png”, you can create three resource files called “logo16.res”, “logo32.res”, and “logo64.res”. Each file should contain the same image, but resized to 16×16, 32×32, and 64×64 pixels respectively.
Step 2: Add the resource files to your project
In Delphi, go to Project > Add to Project > Resource file and select each of the .res files you created in Step 1.
Step 3: Add a TMultiResBitmap component to your form
In the Delphi IDE, go to the Component Palette and find the TMultiResBitmap component. Drag and drop it onto your form.
Step 4: Set the resource files for the TMultiResBitmap component
Select the TMultiResBitmap component on your form and go to the Object Inspector. Click on the Bitmaps property and add a new bitmap for each of the resource files you created in Step 1. For example:
Bitmaps = <
item
Name = 'Logo16'
ResourceName = 'logo16'
end
item
Name = 'Logo32'
ResourceName = 'logo32'
end
item
Name = 'Logo64'
ResourceName = 'logo64'
end>
Step 5: Add the images to your form
Select the TImage component on your form and go to the Object Inspector. Set the MultiResBitmap property to the TMultiResBitmap component you added in Step 3. Then, set the ImageIndex property to the index of the image you want to use. For example, to use the 32×32 version of the logo, set ImageIndex to 1 (since the first image has an index of 0).
Step 6: Access the images in code
To access the images in code, use the TMultiResBitmap.GetBitmap method. For example:
Image1.Picture.Bitmap := MultiResBitmap1.GetBitmap('Logo32');
This code will set the picture of Image1 to the 32×32 version of the logo.
Here’s the updated code for the Delphi form that uses multilanguage pictures with TMultiLang and TMultiResBitmap:
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, MultiLang, MultiResBitmap, StdCtrls, ExtCtrls;
type
TForm1 = class(TForm)
MultiLang1: TMultiLang;
MultiResBitmap1: TMultiResBitmap;
Image1: TImage;
Label1: TLabel;
Button1: TButton;
procedure Button1Click(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure TForm1.Button1Click(Sender: TObject);
begin
Label1.Caption := MultiLang1.GetText(101);
Image1.Picture.Bitmap := MultiResBitmap1.GetBitmap('Logo32');
end;
end.
Happy Coding!!
Thank you for providing me with these article examples. May I ask you a question? http://www.hairstylesvip.com
Pretty component to content. I just stumbled upon your weblog and in accession capital to assert that I get
in fact enjoyed account your blog posts. Any way I’ll be subscribing
in your feeds or even I success you get right of entry to persistently
fast.
Also visit my webpage; nordvpn coupons inspiresensation (http://url.hys.cz/)
Have you ever thought about adding a little bit more than just your articles?
I mean, what you say is valuable and all.
But think about if you added some great visuals or videos to give your posts
more, “pop”! Your content is excellent but with images and clips,
this blog could undeniably be one of the most beneficial in its field.
Awesome blog!
Feel free to surf to my website: nordvpn coupons inspiresensation –
https://t.co –
I think the admin of this site is actually working hard for his website,
since here every data is quality based data.
Also visit my homepage … nordvpn coupons inspiresensation ()
I just could not go away your web site prior to suggesting that I extremely enjoyed the
standard info an individual supply on your guests?
Is going to be again frequently in order to check up on new
posts
Here is my web site – nordvpn coupons inspiresensation
nordvpn special coupon code 2025 350fairfax
For the reason that the admin of this web page is working, no doubt very quickly it will be well-known, due
to its quality contents.
Good write-up, I’m regular visitor of one’s site, maintain up the excellent operate, and It is going to be a regular visitor for a lengthy time.
My husband and i felt really lucky Emmanuel could deal with his inquiry by way of the precious recommendations he gained through your site. It’s not at all simplistic to just be freely giving helpful tips that many others could have been making money from. We really fully grasp we have you to be grateful to because of that. Those illustrations you have made, the straightforward web site menu, the friendships you can make it possible to create – it is most exceptional, and it’s assisting our son in addition to us imagine that the issue is brilliant, and that’s particularly indispensable. Thank you for all!
Your positive and uplifting words are like a ray of sunshine on a cloudy day Thank you for spreading light and positivity in the world
I have recommended this blog to all of my friends and family It’s rare to find such quality content these days!
This is a great read. Thanks for sharing your insights! And also please SignUp and get 30USD FREE investment plan at https://investurns.com/
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article. https://accounts.binance.com/bg/register?ref=V2H9AFPY
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
I have been exploring for a bit for any high-quality articles or blog posts on this kind of area . Exploring in Yahoo I at last stumbled upon this website. Reading this information So i am happy to convey that I have a very good uncanny feeling I discovered just what I needed. I most certainly will make sure to don抰 forget this site and give it a glance regularly.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
I truly appreciate this post. I have been looking everywhere for this! Thank goodness I found it on Bing. You have made my day! Thank you again
Hi there just wanted to give you a quick heads up. The words in your article seem to be running off the screen in Internet explorer. I’m not sure if this is a formatting issue or something to do with browser compatibility but I thought I’d post to let you know. The design look great though! Hope you get the problem solved soon. Kudos
I love what you guys are usually up too. This type of clever work and exposure! Keep up the very good works guys I’ve added you guys to my blogroll.
Thank you for another excellent article. Where else could anybody get that kind of info in such a perfect way of writing? I’ve a presentation next week, and I’m on the look for such info.
Your article helped me a lot, is there any more related content? Thanks!
You’ve the most impressive websites. http://www.kayswell.com
Great delivery. Outstanding arguments. Keep up the great spirit.
my webpage eharmony special coupon code 2025
I am continually looking online for tips that can help me. Thank you!
Thank you for your articles. http://www.kayswell.com They are very helpful to me. Can you help me with something?
I enjoyed reading your piece and it provided me with a lot of value. http://www.kayswell.com
Great beat ! I would like to apprentice while you amend your web site, http://www.kayswell.com how could i subscribe for a blog site? The account helped me a acceptable deal. I had been a little bit acquainted of this your broadcast provided bright clear concept
Incredible points. Great arguments. Keep up the good effort.
Feel free to surf to my webpage; vpn
Whats up very nice website!! Man .. Excellent .. Wonderful .. I will bookmark your site and take the feeds additionallyKI am satisfied to search out a lot of helpful info right here within the submit, we’d like work out more techniques in this regard, thank you for sharing. . . . . .
Thank you for being of assistance to me. I really loved this article. http://www.kayswell.com
Your articles are extremely helpful to me. Please provide more information! http://www.kayswell.com
I want to thank you for your assistance and this post. It’s been great. http://www.kayswell.com
These jackpots continue to grow until someone wins, typically reaching substantial
quantities. Cleopatra Slots is a 5-reel, 20-payline slot that’s been round since 2012.
If you get a screen full of Cleopatra symbols, it pays
out 200,000 times the bet. It additionally has the Buzz Saw Function, the
Mega Hat Feature, and the Mansion Characteristic.
The varied bonus games imply you both win a big prize outright or have a chance
at different bonus video games. Designed by Light & Marvel, Huff N Puff Slots is in style because it
combines a ninety six.1% expected return with excessive volatility and big jackpots.
You’ll undoubtedly attract the casino’s attention if you start betting excessive.
What constitutes as excessive depends on the casino and its location. In virtually all
instances, you’ll get particular remedy should you deposit at least $100,000.
A Excessive Curler is a term used to explain a gambler who wagers substantial amounts of money,
usually on the highest limits out there at a
casino. High rollers, typically referred to as
“whales,” are the elite of the playing world.
They typically have interaction in high-stakes video games corresponding to poker, blackjack,
baccarat, or roulette, the place the minimum bet is nicely above the
usual limits.
Roll the curler within the paint tray to ensure even distribution of the paint and avoid adding an excessive quantity of paint to the roller.
Before portray, it’s essential to remove any extra lint or fibers that
may have turn out to be caught to the curler nap.
Lint can result in an uneven application of paint, resulting in a less-than-perfect finish.
A lint curler or a vacuum cleaner with a brush attachment can be used to effectively take away lint.
Earlier Than diving into your painting project,
it’s essential to properly prepare your roller for painting.
These rollers offer the benefit of providing an even coat of paint and
reducing the amount of time spent portray.
Your appreciation and respect for the risks of risk have
to be dimmed. In the vernacular, you want nerves of steel and brass cajones, because big wins take big risks to realize.
There cannot be any hesitation or worry of the loss, for the sharks that swim with the whale are in it for the big kill.
Taking a whale down has massive rewards, but the degree at which they’re enjoying is dizzying,
the dangers immeasurable, the rewards immense.
Typically speaking, the payout proportion increases along with the denomination.
You’re attempting to maximise how much money you make per square foot.
How do you just ensure you get lots of cash from both machines?
You improve your common winnings on the lower restrict machine
by setting the payout proportion lower. Nonetheless, whether or not being a excessive curler is definitely value the danger ultimately
is dependent upon the person. The world of high-stakes
gambling could be thrilling, but it additionally carries significant risks.
For those who can navigate this world with grace and fortitude, being a high
curler can be an exhilarating experience.
They accept their lumps, however they negotiate
for the finest possible end result, the best mitigation of their losses.
They own lots; they have lots to lose, but the
world to gain. By the tip, you’ll have a transparent understanding of how to determine on the most effective paint roller on your house project, ensuring a stunning
and skilled finish each time. Excessive stakes players ought
to refer back to this page before selecting a high curler casino.
If you intend on enjoying excessive roller slots online, learn our
casino evaluations to see rankings on the highest US slot
websites for top rollers. Expertise the fun of high-roller slots with exclusive
bonuses if you play on-line at slotsguy.com.
The perks available to excessive rollers are
also better than those supplied to low rollers.
Yes, many on-line casinos supply high-roller slots with giant jackpots and
high RTPs. Look for respected on-line casinos that present unique bonuses for high-stakes players.
High-roller slots offer the highest stakes of any game in a
casino. Most games provide a lot lower payouts than slot machines, so you’re wagering tons of cash for much less of a return. When you play
the slots for giant bets, you’re playing the biggest jackpots at
one of the best odds attainable.
References:
blackcoin
Hey There. I found your blog the usage of msn. This is a really smartly written article. I will be sure to bookmark it and come back to read more of your helpful info. Thank you for the post. I’ll definitely return.
Hello! Someoe in my Facebook group shaed thus website with uss soo I
ccame to ive itt a look. I’m definitely enjoying the information. I’m book-marking
and will bbe tweeting thios to mmy followers!
Wonderful blog aand superb style annd design.
baume a levre cicaplast: PharmaDirecte – pharmacie vendant du viagra sans ordonnance
Have you ever considered publishing an ebook or guest authoring on other blogs?
I have a blog based on the same subjects you discuss and
would really like to have you share some stories/information. I know
my readers would enjoy your work. If you’re even remotely interested,
feel free to shoot me an e-mail.
Your article helped me a lot, is there any more related content? Thanks!
http://snabbapoteket.com/# apotek covidtest
gammal bil nytt apotek: kanyler apotek – apoteka se
badebleie apotek apotek + a-vitamin syre krem apotek
apotek linsvГ¤tska: SnabbApoteket – vape blГҐbГ¤r
http://tryggmed.com/# lus apotek
https://snabbapoteket.shop/# fotmask apotek
snel medicijnen bestellen: Medicijn Punt – medicatie aanvragen
tilbud apotek ryggsmГёrer apotek laktulose apotek
hormonspiral pris apotek: statliga apotek – gravid app frukt
http://zorgpakket.com/# online apotheek recept
svamp tassar hund apotek: se recept apotek – hur stavas yoghurt
kan örontermometer visa för högt SnabbApoteket gummiband tandställning apotek
http://tryggmed.com/# apotek hemoroider
https://tryggmed.com/# influensavaksine 2023 apotek
pharmacy online netherlands: Medicijn Punt – medicijnen online bestellen
covid selvtest apotek: TryggMed – uv drakt baby apotek
http://zorgpakket.com/# mijn medicijnen bestellen
mina recep medicin hemleverans kräm mot pigmentfläckar apotek
medicijen: п»їmedicijnen bestellen – apotheken nederland
apotek online recept: frikort apotek – ashwagandha gravid
http://zorgpakket.com/# apotheken in holland
apteka nl: Medicijn Punt – pseudoephedrine kopen in nederland
apotek Г¶ppet sГ¶ndagar billiga vitaminer vad kostar en hГ¶rapparat
https://snabbapoteket.shop/# fullmakt barn apotek
elektrolytt pulver apotek: TryggMed – ryggsmГёrer apotek
http://tryggmed.com/# apotek åpent langfredag
kolla fГ¶delsemГ¤rken apotek: apotej – hГ¤mta ut recept apotek
brodder boots apotek TryggMed selolje apotek
niederlande apotheke: apotheke nl – online apotheek gratis verzending
https://expresscarerx.online/# ExpressCareRx
https://indiamedshub.com/# IndiaMedsHub
Online medicine order: Online medicine home delivery – world pharmacy india
indian pharmacy IndiaMedsHub online pharmacy india
ExpressCareRx: medi rx pharmacy – provigil india pharmacy
https://medimexicorx.shop/# MediMexicoRx
trusted mexican pharmacy MediMexicoRx order from mexican pharmacy online
online pharmacy india: IndiaMedsHub – IndiaMedsHub
http://expresscarerx.org/# rite aid pharmacy viagra price
https://indiamedshub.com/# reputable indian online pharmacy
п»їlegitimate online pharmacies india: reputable indian pharmacies – IndiaMedsHub
IndiaMedsHub: best india pharmacy – IndiaMedsHub
ExpressCareRx dysfunction priligy johor pharmacy
http://medimexicorx.com/# mexican rx online
Thanks for your help and for writing this post. It’s been great. http://www.hairstylesvip.com
indian pharmacy online: IndiaMedsHub – top 10 online pharmacy in india
https://expresscarerx.online/# ExpressCareRx
buy modafinil from mexico no rx isotretinoin from mexico cheap mexican pharmacy
modafinil mexico online: MediMexicoRx – MediMexicoRx
https://medimexicorx.com/# buying prescription drugs in mexico online
order azithromycin mexico: buy from mexico pharmacy – MediMexicoRx
http://medimexicorx.com/# mexican online pharmacies prescription drugs
cheapest online pharmacy india IndiaMedsHub reputable indian pharmacies
birth control: erectile dysfunction drug – ExpressCareRx
https://medimexicorx.shop/# buying prescription drugs in mexico
mail order pharmacy india: IndiaMedsHub – indian pharmacy online
viagra target pharmacy pharmacy no prescription required clomid mexico pharmacy
american rx pharmacy: celebrex northwest pharmacy – winn dixie pharmacy
https://medimexicorx.com/# MediMexicoRx
http://indiamedshub.com/# IndiaMedsHub
mail order pharmacy india: IndiaMedsHub – best online pharmacy india
ExpressCareRx [url=http://expresscarerx.org/#]ultram pharmacy[/url] ExpressCareRx
IndiaMedsHub: mail order pharmacy india – IndiaMedsHub
https://expresscarerx.online/# cialis india pharmacy
https://sumo.app/forum/tutorials-and-techniques/autonvuokraussivusto
IndiaMedsHub: best online pharmacy india – IndiaMedsHub
gen rx pharmacy cialis pharmacy coupon ExpressCareRx
Great blog right here! Additionally your web site loads up fast!
What web host are you the use of? Can I get your affiliate
hyperlink on your host? I desire my web site loaded up as fast
as yours lol gamefly https://tinyurl.com/2ah5u2sb
https://expresscarerx.org/# online pharmacy no prescription needed
indian pharmacies safe: top online pharmacy india – mail order pharmacy india
https://indiamedshub.shop/# IndiaMedsHub
IndiaMedsHub: india online pharmacy – IndiaMedsHub
telmisartan online pharmacy generic viagra pharmacy reviews trileptal online pharmacy
https://medimexicorx.com/# medication from mexico pharmacy
Bravo, remarkable idea
cheap Zoloft: Zoloft for sale – buy Zoloft online without prescription USA
generic Cialis from India buy Cialis online cheap Tadalafil From India
isotretinoin online: generic isotretinoin – Isotretinoin From Canada
http://finasteridefromcanada.com/# cheap propecia without rx
Thank you for your post. I really enjoyed reading it, especially because it addressed my issue. http://www.kayswell.com It helped me a lot and I hope it will also help others.
purchase generic Zoloft online discreetly: sertraline online – Zoloft Company
cheap Cialis Canada tadalafil online no rx Cialis without prescription
Finasteride From Canada: generic Finasteride without prescription – cheap Propecia Canada
https://tadalafilfromindia.shop/# tadalafil online no rx
https://finasteridefromcanada.com/# buying generic propecia without dr prescription
Accutane for sale: generic isotretinoin – buy Accutane online
https://lexapro.pro/# Lexapro for depression online
generic Finasteride without prescription cheap Propecia Canada generic Finasteride without prescription
buy Cialis online cheap: tadalafil online no rx – Cialis without prescription
cheap Propecia Canada: generic Finasteride without prescription – buy propecia online
https://finasteridefromcanada.com/# order propecia no prescription
Tadalafil From India generic Cialis from India generic Cialis from India
lexapro generic over the counter: buy generic lexapro online – Lexapro for depression online
https://tadalafilfromindia.com/# cheap Cialis Canada
Tadalafil From India: cheap Cialis Canada – Cialis without prescription
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
https://zoloft.company/# buy Zoloft online
Isotretinoin From Canada Isotretinoin From Canada Accutane for sale
Lexapro for depression online: medication lexapro 10 mg – Lexapro for depression online
https://the.hosting/
Lexapro for depression online: Lexapro for depression online – buy lexapro
https://zoloft.company/# purchase generic Zoloft online discreetly
generic sertraline Zoloft Company generic sertraline
generic Finasteride without prescription: Finasteride From Canada – Propecia for hair loss online
http://finasteridefromcanada.com/# cheap Propecia Canada
order isotretinoin from Canada to US: isotretinoin online – generic isotretinoin
https://isotretinoinfromcanada.shop/# cheap Accutane
tadalafil online no rx cheap Cialis Canada tadalafil
isotretinoin online: generic isotretinoin – Accutane for sale
https://finasteridefromcanada.shop/# Finasteride From Canada
Isotretinoin From Canada: generic isotretinoin – Isotretinoin From Canada
Propecia for hair loss online cheap Propecia Canada generic propecia
cheap Propecia Canada: cheap Propecia Canada – cost of cheap propecia without insurance
http://isotretinoinfromcanada.com/# generic isotretinoin
https://tadalafilfromindia.com/# Cialis without prescription
https://ufo.hosting/
Tadalafil From India: buy generic tadalafil 20mg – buy Cialis online cheap
buy Cialis online cheap Cialis without prescription Cialis without prescription
purchase generic Accutane online discreetly: Isotretinoin From Canada – Isotretinoin From Canada
https://zoloft.company/# cheap Zoloft
generic Cialis from India: buy Cialis online cheap – buy Cialis online cheap
cheap Zoloft cheap Zoloft buy Zoloft online without prescription USA
https://tadalafilfromindia.com/# buy Cialis online cheap
generic Finasteride without prescription: cheap Propecia Canada – generic Finasteride without prescription
https://isotretinoinfromcanada.com/# generic isotretinoin
generic sertraline: buy Zoloft online without prescription USA – purchase generic Zoloft online discreetly
https://ping.space/
https://zoloft.company/# buy Zoloft online without prescription USA
Propecia for hair loss online Finasteride From Canada Finasteride From Canada
purchase generic Accutane online discreetly: isotretinoin online – Isotretinoin From Canada
cheap Zoloft: buy Zoloft online – Zoloft Company
https://lexapro.pro/# Lexapro for depression online
Tadalafil From India buy Cialis online cheap tadalafil online no rx
buy Accutane online: order isotretinoin from Canada to US – cheap Accutane
http://tadalafilfromindia.com/# tadalafil online no rx
best price for lexapro Lexapro for depression online Lexapro for depression online
sertraline online: Zoloft for sale – buy Zoloft online
https://the.hosting/es/help/corregir-error-set-password-no-tiene-significado-para-el-usuario-rootlocalhost
cheap Propecia Canada: order cheap propecia – Finasteride From Canada
cheap Zoloft Zoloft Company generic sertraline
Zoloft Company: cheap Zoloft – Zoloft online pharmacy USA
buy Accutane online: purchase generic Accutane online discreetly – order isotretinoin from Canada to US
https://lexapro.pro/# Lexapro for depression online
Zoloft for sale buy Zoloft online Zoloft online pharmacy USA
can you buy lexapro over the counter: buy lexapro online india – п»їlexapro
Tadalafil From India: buy Cialis online cheap – buy Cialis online cheap
Lexapro for depression online generic lexapro australia Lexapro for depression online
buy Zoloft online: Zoloft Company – buy Zoloft online