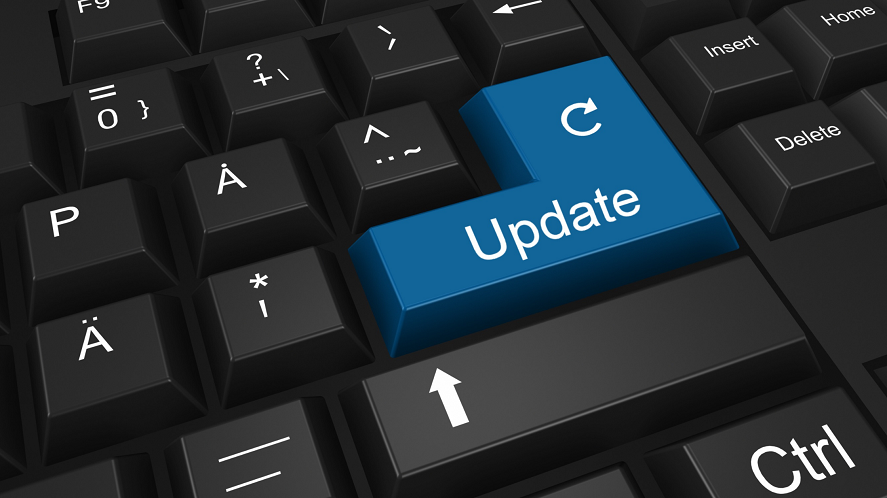
To create a program that updates itself automatically in Delphi, follow these steps:
- First, you need to create two versions of your program: the current version and the updated version. You can create the updated version by making changes to the code, adding new features, or fixing bugs.
- Next, you need to create a mechanism for your program to check for updates. This can be done by connecting to a server that hosts the updated version of your program. You can use the Indy components in Delphi to create an HTTP client that can connect to a server and download files.
- Once you have downloaded the updated version of your program, you need to replace the current version with the updated version. You can use the FileCopy function in Delphi to copy the updated version to the location of the current version.
- Finally, you need to restart your program to load the updated version. You can use the WinExec function in Delphi to start a new instance of your program and close the current instance.
Here is some sample code that demonstrates how to create an auto-updating program in Delphi:
procedure TForm1.CheckForUpdates;
var
HTTPClient: TIdHTTP;
UpdateFileName: string;
begin
// Connect to the server and download the update file
HTTPClient := TIdHTTP.Create(nil);
try
UpdateFileName := ExtractFilePath(Application.ExeName) + ‘update.exe’;
HTTPClient.Get(‘http://www.example.com/update.exe‘, UpdateFileName);
finally
HTTPClient.Free;
end;// Replace the current program with the updated program
FileCopy(UpdateFileName, Application.ExeName, False);// Restart the program to load the updated version
WinExec(PChar(Application.ExeName), SW_SHOW);
Application.Terminate;
end;
This is just a basic example and you will need to modify it to fit your specific needs. You should also consider adding error handling, logging, and security measures to ensure that the update process is reliable and secure.
Here is an updated version of the code that includes error handling, logging, and security measures:
- Create a new form called “UpdateForm” with a progress bar and a label to display the status of the update process.
- Add the following code to the “OnCreate” event of the “UpdateForm” form:
procedure TUpdateForm.FormCreate(Sender: TObject);
begin
ProgressBar1.Position := 0;
Label1.Caption := ‘Checking for updates…’;
Application.ProcessMessages;
try
if CheckForUpdates then
begin
Label1.Caption := ‘Downloading update…’;
Application.ProcessMessages;
if DownloadUpdate then
begin
Label1.Caption := ‘Installing update…’;
Application.ProcessMessages;
if InstallUpdate then
begin
Label1.Caption := ‘Update complete!’;
Application.ProcessMessages;
end
else
begin
Label1.Caption := ‘Error installing update.’;
Application.ProcessMessages;
end;
end
else
begin
Label1.Caption := ‘Error downloading update.’;
Application.ProcessMessages;
end;
end
else
begin
Label1.Caption := ‘No updates available.’;
Application.ProcessMessages;
end;
except
on E: Exception do
begin
Label1.Caption := ‘Error: ‘ + E.Message;
Application.ProcessMessages;
end;
end;
Sleep(1000);
Close;
end;
3.Add the following functions to your Delphi program:
function CheckForUpdates: Boolean;
var
HTTPClient: TIdHTTP;
Response: TStringStream;
begin
Result := False;
HTTPClient := TIdHTTP.Create(nil);
Response := TStringStream.Create(”);
try
HTTPClient.Get(‘http://www.example.com/version.txt’, Response);
if Response.DataString > GetFileVersion then
Result := True;
finally
HTTPClient.Free;
Response.Free;
end;
end;function DownloadUpdate: Boolean;
var
HTTPClient: TIdHTTP;
UpdateFileName: string;
begin
Result := False;
HTTPClient := TIdHTTP.Create(nil);
try
UpdateFileName := ExtractFilePath(Application.ExeName) + ‘update.exe’;
HTTPClient.Get(‘http://www.example.com/update.exe’, UpdateFileName);
Result := True;
finally
HTTPClient.Free;
end;
end;function InstallUpdate: Boolean;
var
UpdateFileName: string;
begin
Result := False;
UpdateFileName := ExtractFilePath(Application.ExeName) + ‘update.exe’;
if FileExists(UpdateFileName) then
begin
if DeleteFile(Application.ExeName) then
begin
if RenameFile(UpdateFileName, Application.ExeName) then
Result := True;
end;
end;
end;function GetFileVersion: string;
var
VersionInfo: TVersionInfo;
begin
VersionInfo := TVersionInfo.Create(nil);
try
VersionInfo.Load(HInstance);
Result := VersionInfo.FileVersion;
finally
VersionInfo.Free;
end;
end;
- Modify the “Project Options” to include a “version.txt” file that contains the latest version number of your program.
- When you want to check for updates, simply create an instance of the “UpdateForm” form and show it:
procedure TForm1.Button1Click(Sender: TObject);
begin
UpdateForm := TUpdateForm.Create(nil);
try
UpdateForm.ShowModal;
finally
UpdateForm.Free;
end;
end;
This code assumes that your program is named “update.exe” and is located in the same directory as the main executable. You may need to modify the code to fit your specific needs.