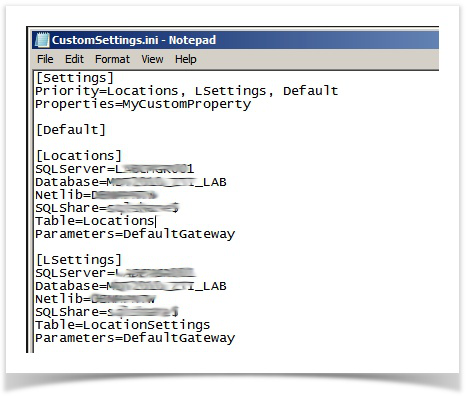
If you’ve been working with Delphi programming for a while, you’ve probably heard of INI files. But what exactly are they, and how can you use them to save and retrieve information in your applications? In this post, we’ll explore the basics of INI files and show you how to work with them in Delphi.
So, what is an INI file? At its simplest, an INI file is a plain text file that contains configuration information for an application. It’s called an INI file because it typically has a “.ini” file extension, although this is not strictly necessary. INI files are often used to store application settings that the user can modify, such as window positions, font choices, and other preferences.
To save information to an INI file in Delphi, you can use the TIniFile class from the IniFiles unit. Here’s an example of how to save some settings to an INI file:
uses IniFiles;
var
IniFile: TIniFile;
begin
IniFile := TIniFile.Create(‘settings.ini’);
try
IniFile.WriteString(‘Window’, ‘Title’, ‘My Application’);
IniFile.WriteInteger(‘Window’, ‘Width’, 800);
IniFile.WriteInteger(‘Window’, ‘Height’, 600);
finally
IniFile.Free;
end;
end;
In this code, we create a new TIniFile object and specify the name of the INI file we want to use. We then use the WriteString and WriteInteger methods to save some settings to the INI file. The first parameter of these methods specifies the section of the INI file where the setting should be saved, and the second parameter specifies the name of the setting. The third parameter is the value to be saved.
To read information from an INI file in Delphi, you can use the same TIniFile class. Here’s an example of how to read the settings we just saved:
uses IniFiles;
var
IniFile: TIniFile;
Title: string;
Width, Height: Integer;
begin
IniFile := TIniFile.Create(‘settings.ini’);
try
Title := IniFile.ReadString(‘Window’, ‘Title’, ‘Default Title’);
Width := IniFile.ReadInteger(‘Window’, ‘Width’, 640);
Height := IniFile.ReadInteger(‘Window’, ‘Height’, 480);
finally
IniFile.Free;
end;
// Now do something with the settings we just read…
end;
In this code, we create a new TIniFile object and again specify the name of the INI file we want to use. We then use the ReadString and ReadInteger methods to retrieve the settings we previously saved. The first two parameters of these methods are the same as for the WriteString and WriteInteger methods (i.e., the section and setting names), while the third parameter specifies a default value to use if the setting is not found in the INI file.
When you’re storing sensitive data in an INI file, such as login credentials or other private information, you may want to encrypt the data so that it can’t be easily read if someone opens the file. One way to do this is to use a simple encryption algorithm to scramble the data before writing it to the INI file, and then unscramble it when reading it back.
Here’s an example of how you could modify the previous code to encrypt the data using a basic XOR encryption algorithm:
uses
IniFiles, SysUtils;
const
IniFileName = ‘settings.ini’;
EncryptionKey = $3B; // change this to your own encryption key
var
IniFile: TIniFile;
function Encrypt(const Input: string): string;
var
I: Integer;
begin
SetLength(Result, Length(Input));
for I := 1 to Length(Input) do
Result[I] := Char(Byte(Input[I]) xor EncryptionKey);
end;
function Decrypt(const Input: string): string;
begin
Result := Encrypt(Input); // XOR encryption is symmetrical
end;
procedure SaveSettings;
begin
IniFile := TIniFile.Create(IniFileName);
try
IniFile.WriteString(‘Window’, ‘Title’, Encrypt(‘My Application’));
IniFile.WriteInteger(‘Window’, ‘Width’, Encrypt(800));
IniFile.WriteInteger(‘Window’, ‘Height’, Encrypt(600));
finally
IniFile.Free;
end;
end;
procedure LoadSettings;
var
Title: string;
Width, Height: Integer;
begin
IniFile := TIniFile.Create(IniFileName);
try
Title := Decrypt(IniFile.ReadString(‘Window’, ‘Title’, ‘Default Title’));
Width := Decrypt(IniFile.ReadInteger(‘Window’, ‘Width’, 640));
Height := Decrypt(IniFile.ReadInteger(‘Window’, ‘Height’, 480));
finally
IniFile.Free;
end;
// Now do something with the settings we just read…
end;
In this code, we define a constant called “EncryptionKey” that serves as the secret key for our XOR encryption algorithm. We then define two helper functions called “Encrypt” and “Decrypt” that use this key to scramble and unscramble the data, respectively.
To encrypt the data before saving it to the INI file, we simply call the Encrypt function on each value we want to save. To read the data back from the INI file, we call the Decrypt function on each value we retrieve.
Of course, this basic encryption algorithm is not very secure and could be easily broken by someone who knows what they’re doing. If you need stronger encryption for your INI files, you should consider using a more robust encryption algorithm, such as AES or RSA. There are libraries available for Delphi that can help you implement these algorithms.
With these basic techniques, you can easily save and retrieve information from INI files in your Delphi applications. Of course, this is just the tip of the iceberg when it comes to working with INI files – there are many other options and features you can use to customize your INI file handling to suit your specific needs. But hopefully this post has given you a good starting point for exploring the world of INI files in Delphi!