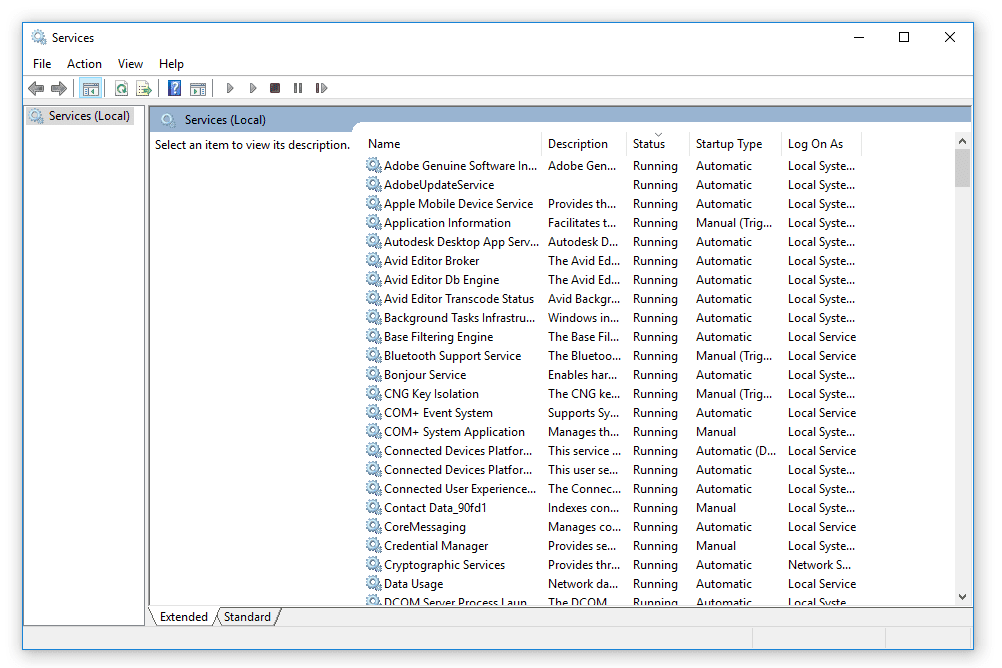
Even when no user is logged in to a Windows operating system, Windows Services continue to execute in the background. They are frequently employed for jobs that call for extended operations, such as running servers or keeping track of system events. In this article, we’ll look at using Windows Services in the Delphi programming language, give some usage examples, and talk about their benefits and drawbacks.
How to Use Windows Services in Delphi
The TService class offered by the Delphi library can be used to establish a Windows Service in Delphi. The ServiceStart and ServiceStop methods that are necessary for creating a Windows Service are included in this class and are called when the service is started and ended, respectively.
To create a new Windows Service project in Delphi, you can follow these steps:
- Open Delphi and choose “File” > “New” > “Other” > “Service Application”.
- In the “Service Application” dialog box, enter a name and description for your service.
- In the “Service Components” section, add any additional components that your service requires. For example, if your service needs to interact with a database, you can add a TADOConnection component.
- In the “ServiceStart” method, add the code your service needs to run when it is started. This might include setting up database connections, initializing variables, and starting timers.
- In the “ServiceStop” method, add the code that your service needs to run when it is stopped. This might include closing database connections, stopping timers, and releasing resources.
- Once you have written your code, you can install your service by choosing “Project” > “Install Service”.
Examples of Windows Services in Delphi
Here are some examples of how you can use Windows Services in Delphi:
- Server applications: You can use a Windows Service to create a server application that runs in the background. For example, you might create a service that listens for incoming connections and responds to client requests.
- Background tasks: You can use a Windows Service to perform background tasks that need to run continuously. For example, you might create a service that monitors system events and logs them to a file.
- Scheduled tasks: You can use a Windows Service to perform scheduled tasks. For example, you might create a service that runs a backup script every night at midnight.
Advantages and Disadvantages of Windows Services
Advantages:
- Background execution: Windows Services can run in the background without requiring any user interaction.
- Reliability: Windows Services are designed to be reliable and can automatically restart if they encounter an error.
- Security: Windows Services can be run under a separate user account, which can help to increase security.
Disadvantages:
- Complexity: Windows Services can be more complex to develop than other types of applications, as they require specialized knowledge and skills.
- Debugging: Debugging a Windows Service can be more difficult than debugging other types of applications, as it is not possible to interact with the service directly.
- Resource usage: Windows Services can consume many system resources if they are not properly optimized, which can cause performance issues.
Here’s an example of how to create a Windows Service in Delphi using the TService class:
- Open RAD Studio and create a new “Service Application” project.
- In the Project Manager, right-click on the “Project1” item and select “Add New” > “Service” from the context menu.
- In the “New Service” dialog box, enter a name for your service (e.g. “MyService”) and click “OK”.
- In the “MyService.pas” file created, you will see a default implementation of the TService class. You can add your own code to the ServiceStart and ServiceStop methods to customize the behavior of your service. For example:
unit MyService;
interface
uses
Winapi.Windows, Winapi.Messages, System.SysUtils, System.Classes, Vcl.SvcMgr;
type
TMyService = class(TService)
procedure ServiceStart(Sender: TService; var Started: Boolean);
procedure ServiceStop(Sender: TService; var Stopped: Boolean);
private
{ Private declarations }
public
function GetServiceController: TServiceController; override;
{ Public declarations }
end;
var
MyService: TMyService;
implementation
{$R *.dfm}
procedure ServiceController(CtrlCode: DWord); stdcall;
begin
MyService.Controller(CtrlCode);
end;
function TMyService.GetServiceController: TServiceController;
begin
Result := ServiceController;
end;
procedure TMyService.ServiceStart(Sender: TService; var Started: Boolean);
begin
// Add your code here to initialize your service
// For example, you might start a timer or open a database connection
Started := True;
end;
procedure TMyService.ServiceStop(Sender: TService; var Stopped: Boolean);
begin
// Add your code here to stop your service
// For example, you might stop a timer or close a database connection
Stopped := True;
end;
end.
- To install your service, right-click on the “MyService” item in the Project Manager and select “Install”. This will register your service with the Windows Service Control Manager and start it running in the background.
I’m done now! A fundamental Windows Service is now active on your system. Keep in mind that you can further personalize your service by adding any parts and programs you require to the ServiceStart and ServiceStop procedures.
Conclusion
In Windows operating systems, Windows Services can be a strong tool for carrying out background operations. Thanks to the TService class offered by the Delphi library, building a Windows Service in Delphi is rather simple. However, in order to prevent performance problems, it’s crucial to properly optimize your code and be aware of the benefits and drawbacks of using Windows Services.