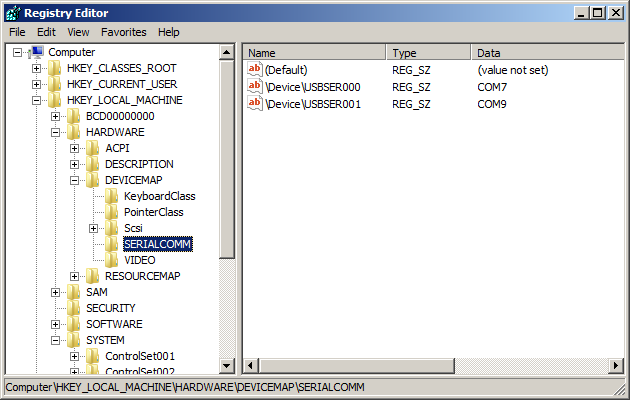
The Windows Registry is a powerful tool that can be used to store and retrieve application settings and data. In this blog post, we’ll explore how we can use the registry in Delphi programming to seed data, withdraw data when the program is started, and protect sensitive information from prying eyes.
Seeding data in the registry One of the most common uses of the registry is to store application settings that can be accessed across multiple sessions. For example, if you want to remember the last path that the user opened a file from, you can store this information in the registry. To do this in Delphi, you can use the TRegistry class from the Registry unit:
uses
Registry;
procedure SaveLastPath(const APath: string);
var
Reg: TRegistry;
begin
Reg := TRegistry.Create;
try
Reg.RootKey := HKEYCURRENTUSER;
if Reg.OpenKey(‘Software\MyApp’, True) then
begin
Reg.WriteString(‘LastPath’, APath);
Reg.CloseKey;
end;
finally
Reg.Free;
end;
end;
In this example, we create a new instance of TRegistry, set the RootKey to HKEYCURRENTUSER, and open the key ‘Software\MyApp’ (which will be created if it doesn’t already exist) with the OpenKey method. We then write the last path to the registry using the WriteString method and close the key with the CloseKey method.
Withdrawing data from the registry Once we’ve stored data in the registry, we can retrieve it when the program starts up. For example, if we want to set the initial directory for the open dialog to the last path that the user opened a file from, we can read this information from the registry. To do this in Delphi, we can use the same TRegistry class:
function GetLastPath: string;
var
Reg: TRegistry;
begin
Result := ”;
Reg := TRegistry.Create;
try
Reg.RootKey := HKEYCURRENTUSER;
if Reg.OpenKey(‘Software\MyApp’, False) then
begin
Result := Reg.ReadString(‘LastPath’);
Reg.CloseKey;
end;
finally
Reg.Free;
end;
end;
In this example, we create a new instance of TRegistry, set the RootKey to HKEYCURRENTUSER, and open the key ‘Software\MyApp’ (which should exist) with the OpenKey method. We then read the last path from the registry using the ReadString method and close the key with the CloseKey method.
Protecting sensitive information in the registry Sometimes, we may need to store sensitive information in the registry, such as passwords or API keys. To protect this information from prying eyes, we can encrypt it before writing it to the registry and decrypt it when reading it back. To do this in Delphi, we can use the TRegistry class along with the EncryptString and DecryptString functions from the SysUtils unit:
function Encrypt(const AValue: string): string;
begin
Result := EncryptString(AValue);
end;
function Decrypt(const AValue: string): string;
begin
Result := DecryptString(AValue);
end;
procedure SavePassword(const APassword: string);
var
Reg: TRegistry;
begin
Reg := TRegistry.Create;
try
Reg.RootKey := HKEYCURRENTUSER;
if Reg.OpenKey(‘Software\MyApp’, True) then
begin
Reg.WriteString(‘Password’, Encrypt(APassword));
Reg.CloseKey;
end;
finally
Reg.Free;
end;
end
In this example, we define two helper functions: Encrypt and Decrypt. EncryptString and DecryptString are built-in functions in Delphi that use the Windows Data Protection API (DPAPI) to encrypt and decrypt strings, respectively. We can then use these functions to encrypt the password before writing it to the registry and decrypt it when reading it back.
To read the encrypted password from the registry, we can modify the GetLastPath function to include the Decrypt function:
function GetPassword: string;
var
Reg: TRegistry;
begin
Result := ”;
Reg := TRegistry.Create;
try
Reg.RootKey := HKEYCURRENTUSER;
if Reg.OpenKey(‘Software\MyApp’, False) then
begin
Result := Decrypt(Reg.ReadString(‘Password’));
Reg.CloseKey;
end;
finally
Reg.Free;
end;
end;
In this example, we read the encrypted password from the registry using the ReadString method and pass it to the Decrypt function to get the original password.
In conclusion, the Windows Registry is a powerful tool that can be used to store and retrieve application settings and data in Delphi programming. By using the TRegistry class and some helper functions, we can easily seed data, withdraw data when the program is started, and protect sensitive information from prying eyes.
- Use constants or variables to store registry keys and values Instead of hardcoding registry keys and values in your code, it’s a good practice to use constants or variables to make it easier to change them later. For example:
const
MyAppRegistryKey = ‘Software\MyApp’;
LastPathValue = ‘LastPath’;
procedure SaveLastPath(const APath: string);
var
Reg: TRegistry;
begin
Reg := TRegistry.Create;
try
Reg.RootKey := HKEYCURRENTUSER;
if Reg.OpenKey(MyAppRegistryKey, True) then
begin
Reg.WriteString(LastPathValue, APath);
Reg.CloseKey;
end;
finally
Reg.Free;
end;
end;
In this example, we define two constants, MyAppRegistryKey and LastPathValue, to store the registry key and value names, respectively. This makes it easier to change these values in the future without having to update all instances of the hardcoded strings in your code.
- Use try-finally blocks to ensure registry keys are properly closed When working with the registry in Delphi, it’s important to properly close registry keys after you’re done using them to prevent memory leaks and other issues. To ensure that registry keys are always properly closed, you can use a try-finally block like this:
var
Reg: TRegistry;
begin
Reg := TRegistry.Create;
try
// Open registry key and do some operations
finally
Reg.Free;
end;
end;
In this example, we create a new instance of TRegistry, open the registry key, and perform some operations. The finally block ensures that the registry key is always closed, even if an exception is thrown.
- Consider using alternative data storage options for large or complex data While the Windows Registry can be a useful tool for storing small amounts of application data, it may not be the best choice for large or complex data structures. In these cases, you may want to consider using alternative data storage options such as a database, XML or JSON files, or even a custom binary file format.
By considering these suggestions, you can use the Windows Registry in Delphi programming effectively and efficiently to store and retrieve application settings and data.