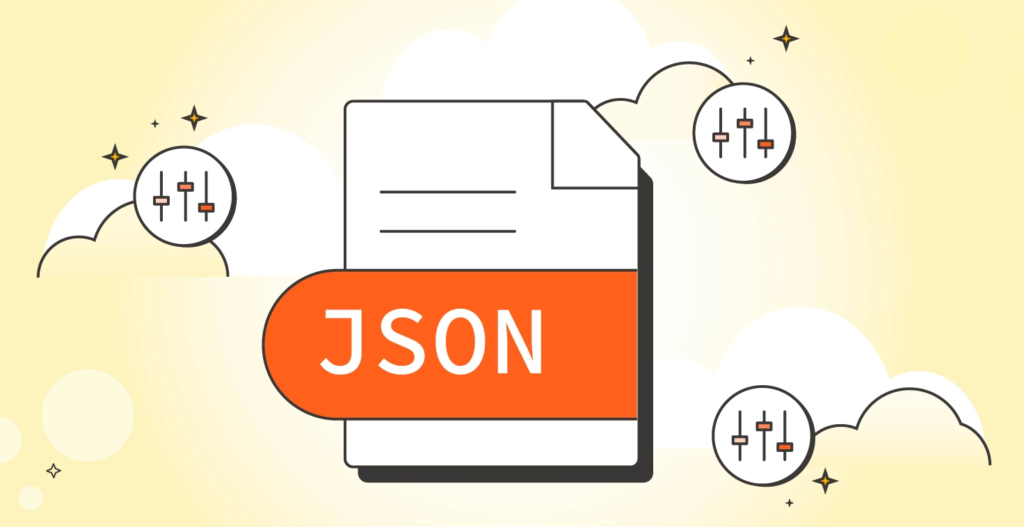
As more developers look to create programs that can run on several operating systems, cross-platform application development has grown in popularity. In cross-platform development, controlling application settings is a crucial step. With some helpful components and examples, we’ll go over how to utilize JSON to store application settings in Delphi in this post.
What is JSON?
JSON (JavaScript Object Notation) is a simple data exchange format that is simple for both humans and machines to read, write, parse, and produce. Although it is built on a portion of JavaScript, it is not dependent on JavaScript to function.
JSON is frequently used in web applications to store configuration information and other settings, but it may also be utilized in desktop and mobile apps. JSON can be used in Delphi to store application settings that are simple to read and write on several platforms.
Using JSON to Store Application Settings in Delphi
You must use a JSON library or component in Delphi to use JSON to store application settings. SuperObject, DelphiJSON, and mORMot are just a few of the free and open source JSON components that are readily available for Delphi.
After selecting a JSON component, you can begin storing and retrieving application settings using it. Here is an illustration of how to use SuperObject to store and retrieve application settings:
uses
SuperObject;
procedure SaveSettings;
var
Settings: ISuperObject;
begin
Settings := TSuperObject.Create;
Settings.S[‘Username’] := ‘John’;
Settings.S[‘Password’] := ‘mypassword’;
Settings.I[‘Port’] := 8080;
Settings.B[‘RememberMe’] := True;
Settings.SaveTo(‘settings.json’);
end;
procedure LoadSettings;
var
Settings: ISuperObject;
begin
Settings := TSuperObject.Create;
Settings.LoadFromFile(‘settings.json’);
ShowMessage(Settings.S[‘Username’]);
ShowMessage(Settings.S[‘Password’]);
ShowMessage(IntToStr(Settings.I[‘Port’]));
ShowMessage(BoolToStr(Settings.B[‘RememberMe’], True));
end;
In this illustration, the application settings are stored in a brand-new TSuperObject. A username, password, port number, and a boolean flag to remember the user were then set as values for the settings. The settings are then saved to a file called settings.json.
We import the settings.json file into a new TSuperObject to retrieve the settings, and then we use the S, I, and B properties to get the settings’ values.
Free JSON Components for Delphi
To save and retrieve application settings, you can use the following free JSON components for Delphi:
A quick and efficient JSON library for Delphi called SuperObject.
DelphiJSON is a straightforward and user-friendly JSON library for Delphi.
mORMot: A robust and adaptable open source Delphi framework that supports JSON.
Example 1: Reading JSON Data from a URL
You can use Delphi’s TNetHTTPClient
component to retrieve JSON data from a URL. Here’s an example of how to retrieve JSON data from the OpenWeatherMap API:
uses
System.Net.HttpClient, System.JSON;
procedure GetWeatherData;
var
Client: TNetHTTPClient;
Response: IHTTPResponse;
JSONData: TJSONObject;
begin
Client := TNetHTTPClient.Create(nil);
try
Response := Client.Get(‘https://api.openweathermap.org/data/2.5/weather?q=New%20York&appid=YOURAPPID’);
if Response.StatusCode = 200 then
begin
JSONData := TJSONObject.ParseJSONValue(Response.ContentAsString) as TJSONObject;
ShowMessage(JSONData.GetValue(‘name’).Value);
ShowMessage(JSONData.Get(‘weather’).JsonValue.ToString);
ShowMessage(JSONData.Get(‘main’).GetValue(‘temp’).Value);
end;
finally
Client.Free;
end;
end;
In this example, we use TNetHTTPClient
to retrieve JSON data from the OpenWeatherMap API. We then parse the JSON data using Delphi’s built-in TJSONObject
class and display some of the data in a message box.
Example 2: Writing JSON Data to a File
You can use Delphi’s TStreamWriter
component to write JSON data to a file. Here’s an example of how to create a JSON file with some sample data:
uses
System.JSON, System.Classes;
procedure WriteJSONToFile;
var
Writer: TStreamWriter;
JSONData: TJSONObject;
begin
JSONData := TJSONObject.Create;
JSONData.AddPair(‘name’, ‘John’);
JSONData.AddPair(‘age’, TJSONNumber.Create(30));
JSONData.AddPair(‘isMarried’, TJSONBool.Create(True));
Writer := TStreamWriter.Create(‘sample.json’);
try
Writer.Write(JSONData.ToString);
finally
Writer.Free;
end;
end;
In this example, we create a new TJSONObject
with some sample data (a name, age, and marital status). We then create a new TStreamWriter
to write the JSON data to a file called sample.json
.
Example 3: Executing a JSON-RPC Call
JSON-RPC is a remote procedure call (RPC) protocol encoded in JSON. You can use Delphi’s TIdHTTP
component to execute a JSON-RPC call. Here’s an example of how to execute a JSON-RPC call using the Blockchain.info API:
uses
IdHTTP, IdGlobal, System.JSON;
procedure ExecuteJSONRPC;
var
IdHTTP: TIdHTTP;
JSONRequest: TJSONObject;
JSONResponse: TJSONObject;
ResponseString: string;
begin
IdHTTP := TIdHTTP.Create(nil);
try
JSONRequest := TJSONObject.Create;
JSONRequest.AddPair(‘method’, ‘blockchain.info/latestblock’);
JSONRequest.AddPair(‘params’, TJSONArray.Create);
ResponseString := IdHTTP.Post(‘https://blockchain.info/‘, JSONRequest.ToString, IndyTextEncoding_UTF8);
JSONResponse := TJSONObject.ParseJSONValue(ResponseString) as TJSONObject;
ShowMessage(JSONResponse.GetValue(‘hash’).Value);
finally
IdHTTP.Free;
end;
end;
In this example, we use TIdHTTP
to execute a JSON-RPC call to the Blockchain.info API. We build a new JSON request using Delphi’s TJSONObject
class and add the method
and params
values. We then use TIdHTTP
to post the JSON request to the API and receive a response. Finally, we parse the JSON response using TJSONObject.ParseJSONValue
and display some of the data in a message box.
These are just a few examples of how to read, write, and execute JSON in Delphi. There are many more use cases for JSON, including serialization, deserialization, and data storage. To work with JSON in Delphi, you can use built-in classes such as TJSONObject
and TJSONValue
, as well as third-party libraries like SuperObject and mORMot.
Conclusion
Managing cross-platform development is made simple and efficient by using JSON to store application parameters in Delphi. You may quickly store and retrieve application settings in JSON format with the aid of free and open source JSON components like SuperObject, DelphiJSON, and mORMot.