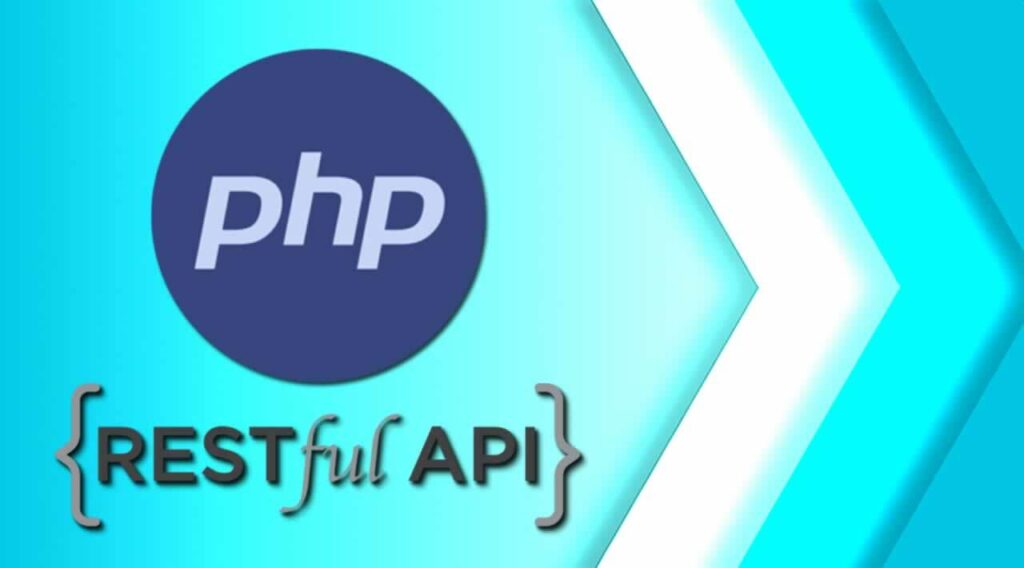
Building RESTful APIs has become an essential component of web development in today’s linked society. REST (Representational State Transfer) is a design pattern that allows clients to interact with web services through basic HTTP methods. In this post, we’ll look at how to build a RESTful API with PHP, as well as examples of authentication, GET, PUT, DELETE, and SELECT operations.
- Establishing the Project:
To begin, ensure that PHP is installed on your PC. Make a new project directory and navigate to it from the command line. Now, let’s create a new PHP project with Composer, a popular PHP dependency manager:
$ composer init
Follow the prompts to provide necessary project details and dependencies.
- Installing Required Libraries: Next, we need to install some libraries to help us build the RESTful API. In this example, we’ll use the Slim Framework, a lightweight and powerful PHP framework for creating APIs:
$ composer require slim/slim
- Creating the API Endpoints: Now, let’s create the API endpoints for our example. We’ll focus on a simple “tasks” API with the following operations: authentication, GET (retrieve tasks), PUT (update a task), DELETE (delete a task), and SELECT (retrieve a specific task).
<?php
use Psr\Http\Message\ResponseInterface as Response;
use Psr\Http\Message\ServerRequestInterface as Request;
use Slim\Factory\AppFactory;
require DIR . ‘/vendor/autoload.php’;
$app = AppFactory::create();
// Sample authentication endpoint
$app->post(‘/login’, function (Request $request, Response $response, $args) {
// Perform authentication logic here
// Return appropriate response
});
// Retrieve all tasks
$app->get(‘/tasks’, function (Request $request, Response $response, $args) {
// Fetch all tasks from the database or any other data source
// Return JSON response with tasks
});
// Update a task
$app->put(‘/tasks/{id}’, function (Request $request, Response $response, $args) {
$taskId = $args[‘id’];
// Update task with the provided ID
// Return JSON response indicating success or failure
});
// Delete a task
$app->delete(‘/tasks/{id}’, function (Request $request, Response $response, $args) {
$taskId = $args[‘id’];
// Delete task with the provided ID
// Return JSON response indicating success or failure
});
// Retrieve a specific task
$app->get(‘/tasks/{id}’, function (Request $request, Response $response, $args) {
$taskId = $args[‘id’];
// Fetch task with the provided ID
// Return JSON response with the task
});
$app->run();
- Handling Authentication: In the authentication endpoint (
/login
), you can implement your own authentication logic, such as validating credentials against a database or external service. Once authenticated, you can generate and return an access token or session token for subsequent API requests. - Data Source and Database Interaction: To complete the functionality of the API, you’ll need to connect to a database or any other data source. Use appropriate libraries or frameworks like PDO, Eloquent, or Doctrine to perform database operations such as retrieving tasks, updating tasks, deleting tasks, etc.
Congratulations! You’ve learnt the fundamentals of building a RESTful API with PHP. You can quickly process HTTP requests and create powerful APIs using the Slim Framework. To establish trustworthy and robust APIs, remember to secure your endpoints with correct authentication mechanisms and ensure appropriate data processing.
Note: The examples provided here focus on the structure and routing of the API endpoints, but they do not include the actual implementation of the database interactions or authentication logic. You will need to adapt the code to your specific requirements and integrate it with your chosen database or data source.
- Implementing Database Interactions: To interact with a database, you can choose a library or ORM (Object-Relational Mapping) tool based on your preference. Here’s an example of how you can use the PDO library to connect to a MySQL database and perform CRUD operations:
// Add this code to the beginning of your PHP script
// Set up database connection
$dbHost = ‘localhost’;
$dbName = ‘yourdatabasename’;
$dbUser = ‘your_username’;
$dbPass = ‘your_password’;
$dsn = “mysql:host=$dbHost;dbname=$dbName;charset=utf8mb4”;
try {
$db = new PDO($dsn, $dbUser, $dbPass);
$db->setAttribute(PDO::ATTRERRMODE, PDO::ERRMODEEXCEPTION);
} catch (PDOException $e) {
die(“Database connection failed: ” . $e->getMessage());
}
// Retrieve all tasks
$app->get(‘/tasks’, function (Request $request, Response $response, $args) use ($db) {
$query = “SELECT * FROM tasks”;
$stmt = $db->query($query);
$tasks = $stmt->fetchAll(PDO::FETCH_ASSOC);
return $response->withJson($tasks);
});
// Update a task
$app->put(‘/tasks/{id}’, function (Request $request, Response $response, $args) use ($db) {
$taskId = $args[‘id’];
$data = $request->getParsedBody();
$title = $data[‘title’];
$description = $data[‘description’];
$query = “UPDATE tasks SET title = :title, description = :description WHERE id = :id”;
$stmt = $db->prepare($query);
$stmt->bindParam(‘:title’, $title);
$stmt->bindParam(‘:description’, $description);
$stmt->bindParam(‘:id’, $taskId);
$stmt->execute();
return $response->withJson([‘message’ => ‘Task updated successfully’]);
});
// Delete a task
$app->delete(‘/tasks/{id}’, function (Request $request, Response $response, $args) use ($db) {
$taskId = $args[‘id’];
$query = “DELETE FROM tasks WHERE id = :id”;
$stmt = $db->prepare($query);
$stmt->bindParam(‘:id’, $taskId);
$stmt->execute();
return $response->withJson([‘message’ => ‘Task deleted successfully’]);
});
// Retrieve a specific task
$app->get(‘/tasks/{id}’, function (Request $request, Response $response, $args) use ($db) {
$taskId = $args[‘id’];
$query = “SELECT * FROM tasks WHERE id = :id”;
$stmt = $db->prepare($query);
$stmt->bindParam(‘:id’, $taskId);
$stmt->execute();
$task = $stmt->fetch(PDO::FETCH_ASSOC);
return $response->withJson($task);
});
- Securing Endpoints with Authentication: To secure your API endpoints, you can use various authentication mechanisms such as JWT (JSON Web Tokens), OAuth, or session-based authentication. Here’s an example using JWT authentication:
use Firebase\JWT\JWT;
$app->post(‘/login’, function (Request $request, Response $response, $args) use ($db) {
// Retrieve credentials from the request
$data = $request->getParsedBody();
$username = $data[‘username’];
$password = $data[‘password’];
// Validate credentials against the database
$query = “SELECT * FROM users WHERE username = :username AND password = :password”;
$stmt = $db->prepare($query);
$stmt->bindParam(‘:username’, $username);
$stmt->bindParam(‘:password’, $password);
$stmt->execute();
$user = $stmt->fetch(PDO::FETCH_ASSOC);
if (!$user) {
return $response->withJson([‘error’ => ‘Invalid credentials’], 401);
}
// Generate JWT token
$payload = [‘user_id’ => $user[‘id’]];
$token = JWT::encode($payload, ‘yoursecretkey’);
return $response->withJson([‘token’ => $token]);
});
// Protected route: retrieve all tasks
$app->get(‘/tasks’, function (Request $request, Response $response, $args) use ($db) {
// Verify JWT token
$token = $request->getHeaderLine(‘Authorization’);
try {
$decodedToken = JWT::decode($token, ‘yoursecretkey’, [‘HS256’]);
} catch (Exception $e) {
return $response->withJson([‘error’ => ‘Invalid token’], 401);
}
// Fetch tasks from the database
$query = “SELECT * FROM tasks”;
$stmt = $db->query($query);
$tasks = $stmt->fetchAll(PDO::FETCH_ASSOC);
return $response->withJson($tasks);
});
Please note that the above examples assume the installation of additional libraries like `Firebase\JWT\JWT` for JWT token handling. Make sure to include the necessary require
statements at the beginning of your PHP script.
By following the steps outlined in this guide, you can create a RESTful API with PHP. We covered setting up the project, installing required libraries, creating API endpoints, implementing database interactions, and securing the endpoints with authentication. Remember to adapt the code examples to fit your specific needs and integrate them with your chosen database or data source.
Here’s an example of how you can consume the RESTful API endpoints created with PHP in a Delphi programming language.
- Make sure you have the Delphi IDE installed on your machine.
- Create a new Delphi project.
- Add the required components to your project:
- TIdHTTP: Used for making HTTP requests.
- TJSONObject: Used for parsing JSON responses.
- In your Delphi code, you can use the TIdHTTP component to interact with the RESTful API. Here’s an example of consuming the GET request to retrieve all tasks:
uses
System.JSON, IdHTTP;
procedure RetrieveAllTasks;
var
HTTPClient: TIdHTTP;
Response: string;
JSONTasks: TJSONObject;
begin
HTTPClient := TIdHTTP.Create;
try
Response := HTTPClient.Get(‘http://example.com/tasks‘);
JSONTasks := TJSONObject.ParseJSONValue(Response) as TJSONObject;
// Handle the JSON response
// Access and process the tasks retrieved from the API
// …
finally
JSONTasks.Free;
HTTPClient.Free;
end;
end;
- Similarly, you can consume other endpoints like PUT, DELETE, and SELECT by modifying the HTTP request accordingly. Here’s an example of making a PUT request to update a task:
uses
IdHTTP;
procedure UpdateTask(taskId: Integer; title, description: string);
var
HTTPClient: TIdHTTP;
RequestJSON: string;
begin
HTTPClient := TIdHTTP.Create;
try
RequestJSON := Format(‘{“title”: “%s”, “description”: “%s”}’, [title, description]);
HTTPClient.Put(‘http://example.com/tasks/‘ + IntToStr(taskId), RequestJSON);
// Handle the response
// …
finally
HTTPClient.Free;
end;
end;
- Remember to handle exceptions, error responses, and any additional processing logic based on your application’s requirements.
- Repeat the above steps for other HTTP methods (POST, DELETE, etc.) and endpoints as needed.
Note: Replace 'http://example.com'
with the actual base URL of your RESTful API.
Make sure to customize the code according to your specific needs, such as handling the JSON response, error checking, and updating the UI or data structures in your Delphi application accordingly.
To add authentication to your Delphi application before accessing other APIs, you can follow these steps:
- Implement the authentication process in your Delphi application. This typically involves collecting the user’s credentials (e.g., username and password) and sending them to the authentication endpoint of your PHP API.
uses
IdHTTP, System.JSON;
procedure AuthenticateUser(username, password: string);
var
HTTPClient: TIdHTTP;
RequestJSON: string;
Response: string;
AccessToken: string;
begin
HTTPClient := TIdHTTP.Create;
try
RequestJSON := Format(‘{“username”: “%s”, “password”: “%s”}’, [username, password]);
Response := HTTPClient.Post(‘http://example.com/login‘, RequestJSON);
// Parse the JSON response to retrieve the access token
AccessToken := TJSONObject.ParseJSONValue(Response).GetValue<string>(‘token’);
// Store the access token for subsequent API requests
// …
finally
HTTPClient.Free;
end;
end;
- Once the user is authenticated and you have obtained the access token, you can include it in the headers of subsequent API requests. The exact method for including headers may vary based on the HTTP client library you are using. Here’s an example using TIdHTTP:
procedure RetrieveAllTasks;
var
HTTPClient: TIdHTTP;
Response: string;
begin
HTTPClient := TIdHTTP.Create;
try
// Set the access token in the ‘Authorization’ header
HTTPClient.Request.CustomHeaders.AddValue(‘Authorization’, ‘Bearer ‘ + AccessToken);
Response := HTTPClient.Get(‘http://example.com/tasks‘);
// Handle the JSON response
// Access and process the tasks retrieved from the API
// …
finally
HTTPClient.Free;
end;
end;
- Make sure to handle exceptions, error responses, and perform proper error checking and handling throughout your application.
Remember to adapt the code to fit your specific requirements and integrate it with your authentication and API endpoints. Additionally, ensure that the authentication mechanism (e.g., JWT tokens) and headers are compatible with the authentication implementation in your PHP API.
Happy coding!